combineReducers
- Redux에서 제공하는 combineReducers 사용
import { combineReducers } from "redux";
const todoApp = combineReducers({
todos,
filter,
});
// src/redux/actions.js
export const ADD_TODO = "ADD_TODO";
export const COMPLETE_TODO = "COMPLETE_TODO";
// { type: ADD_TODO, text: text}
export function addTodo(text) {
return {
type: ADD_TODO,
text,
};
}
// { type: COMPLETE_TODO, index: index }
export function completeTodo(index) {
return {
type: COMPLETE_TODO,
index,
};
}
export const SHOW_ALL = "SHOW_ALL";
export const SHOW_COMPLETE = "SHOW_COMPLETE";
export function showAll() {
return { type: SHOW_ALL };
}
export function showComplete() {
return { type: SHOW_COMPLETE };
}
// src/redux/reducers/reducer.js
import { combineReducers } from "redux";
import todos from "./todos";
import filter from "./filter";
const reducer = combineReducers({
todos,
filter,
});
export default reducer;
// src/redux/reducers/todos.js
import { ADD_TODO, COMPLETE_TODO } from "../actions";
const initialState = [];
export default function todos(previousState = initialState, action) {
if (action.type === ADD_TODO) {
return [...previousState, { text: action.text, done: false }];
}
if (action.type === COMPLETE_TODO) {
return previousState.map((todo, index) => {
if (index === action.index) {
return { ...todo, done: true };
}
return todo;
});
}
return previousState;
}
// src/redux/reducers/filter.js
import { SHOW_COMPLETE, SHOW_ALL } from "../actions";
const initialState = "ALL";
export default function filter(previousState = initialState, action) {
if (action.type === SHOW_COMPLETE) {
return "COMPLETE";
}
if (action.type === SHOW_ALL) {
return "ALL";
}
return previousState;
}
// src/redux/store.js
import { createStore } from "redux";
import todoApp from "./reducers/reducer";
const store = createStore(todoApp);
export default store;
// src/index.js
import React from "react";
import ReactDOM from "react-dom";
import "./index.css";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
import store from "./redux/store";
import { addTodo, completeTodo, showComplete } from "./redux/actions";
store.subscribe(() => {
console.log(store.getState());
});
store.dispatch(addTodo("할일"));
store.dispatch(completeTodo(0));
store.dispatch(showComplete());
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById("root")
);
reportWebVitals();
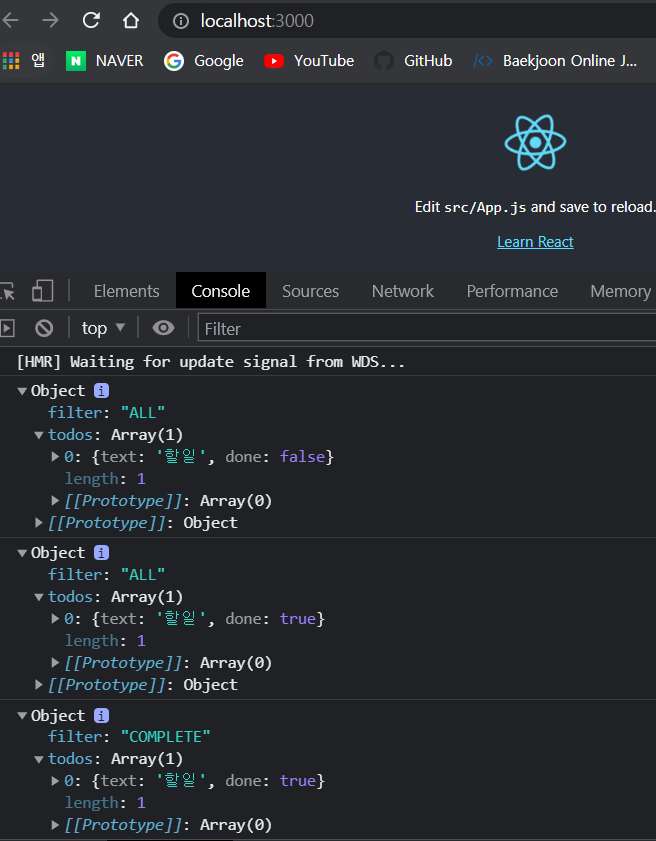
댓글남기기